According to the AJAX paradigm, Web applications work
by exchanging data rather than pages with the Web server. An AJAX page
sends a request with some input arguments and receives a response with
some return values. The code in the client browser orchestrates the
operation, gets the data, and then updates the user interface. From a
user perspective, this means that faster roundtrips occur and, more
importantly, page loading is quicker and the need to refresh the page
entirely is significantly reduced.
As a result, a Web
application tends to look like a classic desktop Microsoft Windows
application. It is allowed to invoke server code from the client and run
and control server-side asynchronous tasks, and it features a strongly
responsive and nonflickering user interface.
Moving Away from Partial Rendering
Partial rendering replaces classic full postbacks with partial
postbacks that update only a portion of the requesting page. An AJAX
postback is more lightweight than a full postback, but a drawback is
that the AJAX postback is still a request that moves view state, event
validation data, and any other input fields you might have around the
page. Also, the AJAX postback is still a request that goes through the
full server-side page life cycle. It differs from a regular ASP.NET
postback only because it has a custom rendering phase and, of course,
returns only a portion of the whole page markup. Put another way, an
AJAX postback is definitely faster and much more beneficial than regular
postbacks, but it’s still subject to a number of constraints. And, more
importantly, it doesn’t fit just any scenario. To fully enjoy the
benefits of AJAX, we have to move past partial rendering and rewrite our
applications to use full-fledged behind-the-scenes asynchronous
communication and user interface updates.
The Flip Side of Partial Rendering
This isn’t to
suggest that partial rendering is inherently bad or wrong. There are two
opposite forces that apply to the Web. One is the force of evolution
signified by AJAX that is geared toward the adoption of new technologies
and patterns. The other is the force of continuity that tends to make
things evolve without disrupting the neat flow from past to present. In
the case of AJAX, the force of continuity is exemplified by ASP.NET
partial rendering.
With
ASP.NET partial rendering, there’s nothing really new architecturally
speaking. It’s just the same ASP.NET model revamped through a set of
tricky solutions that make page rendering smarter and, more importantly,
limited to the fragments of the page that really need a refresh.
Partial rendering
has inherent limitations and should be considered a short-term solution
for adding AJAX capabilities to legacy applications. This said, we can’t
ignore that a significant share of today’s Web applications just need a
bit of facelift to look better and run faster. In this regard, partial
rendering is the perfect remedy.
The ASP.NET AJAX Emerging Model
The ASP.NET application
model based on postback and view state is, technologically speaking,
probably a thing of the past. Of course, this doesn’t mean that
thousands of pages will be wiped out tomorrow and that hundreds of
applications must be rewritten in the upcoming months. More simply, a
superior model for Web applications is coming out that is more powerful,
both technologically and architecturally, and able to serve today’s
demand for enhancing and enriching the user experience.
The AJAX emerging model
is based on two layers—a client –application layer and a server
–application layer. The –client layer sends requests to the –server
layer and the server layer sends back responses. Server endpoints are
identified through URLs and expose data feeds—mostly JavaScript Object
Notation (JSON) data streams—to the client. The –server layer is only a
façade that receives calls and forwards them to the business layer of
the application. Figure 1 depicts the entire model.
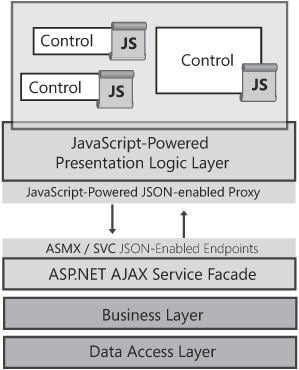
Let’s drill down a bit more in the client layer and –server layer of true AJAX applications for the ASP.NET platform.
Designing the –Client Layer of an ASP.NET AJAX Application
The
–client layer manages the user interface and incorporates the
presentation logic. How would you code the presentation logic? Using
which language or engine? And using which delivery format? For the
client layer to really be cross-browser capable, you should use
JavaScript and HTML. However, by using JavaScript and HTML you can
hardly provide the innovative, immersive, and impressive user experience
that many categories of applications loudly demand.
Limits of JavaScript
JavaScript was not
designed to back up the presentation logic of Web pages. Originally, it
was a small engine added to one of the first versions of Netscape
Navigator with the sole purpose of making HTML pages more interactive.
Today, it is being used for more ambitious tasks, but it’s still nearly
the same relatively simple engine created a decade ago.
JavaScript is an
interpreted, dynamic-binding, and weakly-typed language with first-class
functions. It was influenced by many languages and was designed to look
like a simpler form of Java so that it would be easy to use for
nonexpert Web page authors.
Worse yet, JavaScript is
subject to the browser’s implementation of the engine. The result is
that the same language feature provides different performance on
different browsers and might be flawed on one browser while working
efficiently on others. This limitation makes it difficult to write good,
cross-browser JavaScript code and justifies the love/hate relationship
(well, mostly hate) that many developers have with the language.
Enriching JavaScript
When you move
towards AJAX-based architectures, you basically move some of the
workload to the client. But on the Web, the client is the browser and
JavaScript is currently the sole programming option you have. How can
you get a richer and more powerful JavaScript?
At the end of the
day, JavaScript has two main drawbacks: it is an interpreted language
(significantly slower than a compiled language) and is not fully object
oriented. Extending JavaScript is not as easy and affordable as it might
seem. Being so popular, any radical change to the language risks
breaking a number of applications. But, on the other hand, radical
changes are required to meet the upcoming challenges of AJAX.
There exists a proposed standard for JavaScript 2.0 that is discussed in a paper you can download at http://www.mozilla.org/js/language/evolvingJS.pdf. And at http://developer.mozilla.org/presentations/xtech2006/javascript,
you can read Brendan Eich’s considerations regarding the feature set in
JavaScript 2.0. (Brendan Eich is the inventor of the language.)
It is key to note that
the proposed standard is intended, among other things, to achieve better
support for programs assembled from components and packaged. Time will
tell, however, if
and how JavaScript will undergo a facelift. As a matter of fact, from
the AJAX perspective JavaScript is at the same time a pillar of the Web
but also one of its key bottlenecks.
Using ad hoc libraries
(for example, the Microsoft AJAX library) and widgets (for example,
Dojo, Gaia), you can mitigate some of the JavaScript development issues
and still deploy richer applications. Honestly, there’s not much you can
do to improve the performance of heavyweight JavaScript pages.
The real turning point
for empowering the Web presentation layer is Silverlight 2.0.
Silverlight is a cross-platform browser plug-in that brings a fraction
of the power of the common language runtime (CLR) and.NET Framework to
the browser, including support for managed languages.
Limits of the HTML Markup Language
Today’s Web pages use
HTML to express their contents. But what’s HTML, exactly? Is it a
document format? Or is it rather an application delivery format? Or is
it none of the above? If you look back at the origins of the Web, you
should conclude that HTML is a document format designed to contain
information, some images and, more importantly, links to other
documents.
Today, we use HTML
pages with tables, cascading style sheet (CSS) styles, and lots of
images for the pictures they contain and to add compelling separators
and rounded borders to otherwise ugly and squared blocks of markup. If
you’re looking for a document format, HTML is outdated because it lacks a
number of composing and packaging capabilities that you find, for
example, in the Microsoft Office Open XML formats. If you’re looking for
an application-delivery format, HTML lacks a rich layout model,
built-in graphics, and media capabilities.
Just as for JavaScript,
though, getting rid of HTML is not a decision to make with a light heart
because HTML is popular and used in a wide variety of applications (not
just Web pages). Embedding richer content in a thin HTML wrapper might
be a good compromise. And, again, Silverlight with its full support for
the XAML language and the Windows Presentation Foundation (WPF) engine
is the real turning point.
What About AJAX-Specific Controls?
The programming
model of ASP.NET pages based on server controls gained wide acceptance
and proved to be quite helpful in the past. How are server controls
affected by the aforementioned limitations of JavaScript and HTML, and
what’s the impact of Silverlight on them?
All in all, server
controls are orthogonal to JavaScript, HTML, and even Silverlight.
Server controls are the programming tools used to generate the delivery
format of the application. They can generate HTML as well as XAML
(eXtended Application Markup Language, which is the language of Silverlight), and they can support JavaScript as well as Silverlight or managed languages.
Today, a number of
commercial products exist to take the user interface of Web
applications to the next level. They are all made of a collection of
rich and smart server controls that generate HTML and JavaScript. This
is to say that it’s not by using Telerik or ComponentArt or Infragistics
that you work around the issues that slow down the implementation of
the AJAX paradigm in the today’s Web. Rather, more is required.
A set of lower level
tools is required to enlarge the browser’s capabilities. This can be
obtained in two ways: new browser technology or cross-platform browser
extensions (for example., plug-ins). The first option is a utopian plan
that would take years to be effective. The second option is what you get
with Silverlight.
Designing the –Server Layer of ASP.NET AJAX Applications
There are situations in
which the partial-rendering model is not appropriate and other
situations in which it is just perfect. When the client requires that a
specific operation be executed on the server with no frills and in a
purely stateless manner, you should consider options other than partial
rendering. Enter remote server method calls.
Making a call to a remote
server requires that a public, well-known application programming
interface (API) be exposed and made accessible from JavaScript or
whatever other programming technology you have available in the browser
(for example, Silverlight).
As Figure 1 shows, the –server layer of an AJAX application is made of services. But which services?
A Service-Oriented –Server-Side Architecture
The –server layer is
easy to devise. It is made of services, and on the ASP.NET platform this
can only mean XML Web services or WCF services. Hold on, though. You
should take the preceding statement literally because the involvement of
XML Web services might take you in the wrong direction.
In the context of ASP.NET
AJAX, XML Web services are instrumental to the definition of a public,
contract-based API that JavaScript (or Silverlight) code can invoke. It
doesn’t necessarily mean that you can call just any WS-* Web services
from an AJAX client. In the context of ASP.NET AJAX, I suggest you think
of Web services as a sort of application-specific façade to expose some
server-side logic to a JavaScript (or Silverlight) client.
To be invoked from
within an ASP.NET AJAX page, the remote service must meet a number of
requirements, the strictest of which relate to the location of the
endpoint and underlying platform. AJAX-enabled services must be hosted
in the same domain from which the call is made. This means that a Web service must be an ASP.NET XML Web service (an .asmx endpoint) and must be hosted in an IIS application on the same Web server as the ASP.NET application.
From the client, you
can’t just call any Web services on Earth regardless of location and
platform. This is a security measure; not a technical limitation.
In summary, there are three ways to define services for the –server layer of an ASP.NET AJAX application:
ASP.NET XML Web services with an .asmx endpoint
WCF services with an .svc endpoint
Page methods with an .aspx endpoint defined on the same page that calls them
Note
The
term “service” is a bit overused and often abused. In AJAX, a service
indicates a piece of code that is local to the application (resident on
the domain of the application) and exposes functionalities to the
client. In the end, services used by AJAX applications tend not to use
Simple Object Access Protocol (SOAP) to communicate (they use JSON) and
are not necessarily autonomous services in the service-oriented
architecture (SOA) sense. They are instead bound to the platform and the
domain where they’re hosted. Based on this, they can hardly be called
WS-* Web services or SOA services. |
REST Services
The ideal service for
AJAX applications is centered around the idea of data and resources to
expose to Web clients. It is reachable over HTTP and requires that
clients use URLs (and optionally HTTP headers) to access data and
command operations. Clients interact with the service using HTTP verbs
such as GET, POST, PUT, and DELETE. Put another way, the URL represents a
resource and the HTTP verb describes the action you want to take on the
resource. Data exchanged in those interactions is represented in simple
formats such as JSON and plain XML, or even in syndication formats such
RSS and ATOM.
A service
with these characteristics is a Representational State Transfer (REST)
service. For more information on the definition of REST, have a look at
the original paper that describes the vision behind it. You can find it
at the following URL: http://www.ics.uci.edu/~fielding/pubs/dissertation/top.htm.
Microsoft is currently
working on a new generation of data services for the ASP.NET platform
that fully embodies REST principles. Such data services are slated to be
part of the .NET Framework 3.5 Service Pack 1, which is scheduled to
ship sometime in 2008. Meanwhile, you can get the flavor of it using the latest Community Technology Preview (CTP) of the ASP.NET 3.5 Extensions toolkit.
Data Serialization
Needless to say, the
communication between browser and remote services occurs over the HTTP
protocol. But what about the content of the packets? An AJAX call
consist of some data that is passed as arguments to the invoked service
method and some data that is returned as the output. How is this data
serialized?
The common
serialization format that can be understood on both ends is JavaScript
Object Notation (JSON). You can learn more on syntax and purposes of
JSON at http://www.json.org.
JSON is a
text-based format specifically designed to move the state of an object
across tiers. It is natively supported by JavaScript in the sense that a
JSON-compatible string can be evaluated to a JavaScript object through
the JavaScript eval
function. However, if the JSON string represents the state of a custom
object, it’s your responsibility to ensure that the definition of the
corresponding class is available on the client.
The ASP.NET AJAX
network stack takes care of creating JSON strings for each parameter to
pass remotely. On the server, ad hoc formatter classes receive the data
and use .NET reflection to populate matching managed classes. On the way
back, .NET managed objects are serialized to JSON strings and sent
over. The script manager is called to guarantee that proper classes
referenced in the JSON strings—the Web service proxy class—exist on the
client. The nicest thing is that all this machinery is transparent to
programmers.
The JSON format describes the state of the object as shown here:
{"ID"="ALFKI", "Company":"Alfred Futterkiste"}
The string indicates an object with two properties—ID and Company—and
their respective, text-serialized values. If a property is assigned a
nonprimitive value—say, a custom object—the value is recursively
serialized to JSON.
JSON vs. XML
For years, XML has been
touted as the lingua franca of the Web. Now that AJAX has become a key
milestone for the entire Web, XML is pushed to the side in favor of JSON
as far as data representation over the Web is concerned. Why is that?
Essentially, JSON is
slightly simpler and more appropriate for the JavaScript language than
XML. Although it might be arguable whether JSON is easier to understand
than XML for humans—this is just my thought, by the way—it is certainly
easier than XML for a machine to process. No such thing as an XML parser
is required for JSON. Everything you need to parse the text is built into the JavaScript language. JSON is also less verbose than XML and less ambitious too.
JSON is not
perfect either. The industrial quantity of commas and quotes it requires
makes it a rather quirky format. But can you honestly say that XML is
more forgiving?
With JSON, you also gain a
key architectural benefit at a relatively low cost. You reason in terms
of objects everywhere. On the server, you define your entities and
implement them as classes in your favorite managed language. When a
service method needs to return an instance of any class, the state of
the object is serialized to JSON and travels over the wire. On the
client, the JSON string is received and processed, and its contents are
loaded into an array, or a kind of mirror JavaScript object, with the
same interface as the server class. The interface of the class is
inferred from the JSON stream. In this way, both the service and the
client page code use the same logical definition of an entity—or, more
precisely, of the entity’s data transfer object (DTO).
It goes without saying
that, from a purely technical standpoint, the preservation of the data
contract doesn’t strictly require JSON to be implemented. You could get
to the same point using XML as well. In that case, though, you need to
get yourself an XML parser that can be used from JavaScript.
Parsing some simple XML
text in JavaScript might not be an issue, but getting a full-blown
parser is another story completely. Performance and functionality issues
will likely lead to a proliferation of similar components with little
in common. And then you must decide whether such a JavaScript XML parser
should support things such as namespaces, schemas, whitespaces,
comments, and processing instructions.
As I see it, for the
sake of compatibility you will end up with a subset of XML limited to
nodes and attributes. At that point, it is merely a matter of choosing
between the “angle brackets” of XML and the “curly brackets” of JSON.
Additionally, JSON has a free parser already built into the JavaScript
engine—the aforementioned function eval.